NavigationView: Android Studio
NavigationView is used as a sliding view. It is placed inside a drawer layout. The following dependencies should be implemented in build.gradle(:app). implementation ‘androidx.navigation:navigation-fragment:2.3.3’, androidTestImplementation ‘androidx.test.ext:junit:1.1.2’, androidTestImplementation ‘androidx.test.espresso:espresso-core:3.3.0’. The following code shows NavigationView in a drawer layout with fragments in activity_main.xml.
<?xml version="1.0" encoding="utf-8"?>
<androidx.drawerlayout.widget.DrawerLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/drawerLayout"
tools:context=".MainActivity">
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:id="@+id/topToolBar"
android:layout_width="match_parent"
android:layout_height="?actionBarSize"
android:background="@color/purple_500"
android:gravity="center_vertical"
android:orientation="horizontal"
android:padding="15dp"
app:layout_constraintTop_toTopOf="parent">
<ImageView
android:id="@+id/menuIcon"
android:layout_width="35dp"
android:layout_height="35dp"
android:contentDescription="@string/app_name"
android:src="@drawable/ic_baseline_menu_24"
app:tint="@color/white">
</ImageView>
<TextView
android:id="@+id/title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Menu"
android:textColor="@color/white"
android:textSize="20sp">
</TextView>
</LinearLayout>
<fragment
android:layout_width="match_parent"
android:layout_height="0dp"
app:layout_constraintTop_toBottomOf="@+id/topToolBar"
app:layout_constraintBottom_toBottomOf="parent"
android:id="@+id/nav_fragment"
android:name="androidx.navigation.fragment.NavHostFragment"
app:defaultNavHost="true"
app:navGraph="@navigation/fragment_item">
</fragment>
</androidx.constraintlayout.widget.ConstraintLayout>
<com.google.android.material.navigation.NavigationView
android:id="@+id/navigationView"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="start"
app:menu="@menu/nav_menu"
app:headerLayout="@layout/nav_header_layout">
</com.google.android.material.navigation.NavigationView>
</androidx.drawerlayout.widget.DrawerLayout>
The following code shows MainActivity.java.
package com.example.navigationview;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.view.GravityCompat;
import androidx.drawerlayout.widget.DrawerLayout;
import androidx.navigation.NavController;
import androidx.navigation.Navigation;
import androidx.navigation.ui.NavigationUI;
import android.os.Bundle;
import android.view.View;
import com.google.android.material.navigation.NavigationView;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
DrawerLayout drawerLayout = findViewById(R.id.drawerLayout);
findViewById(R.id.menuIcon).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
drawerLayout.openDrawer(GravityCompat.START);
}
});
NavigationView navigationView = findViewById(R.id.navigationView);
navigationView.setItemIconTintList(null);
NavController navController = Navigation.findNavController(this,R.id.nav_fragment);
NavigationUI.setupWithNavController(navigationView, navController);
}
}
The following code shows navigation resource file fragment_item.xml created in navigation – (android resource directory) in res folder.
<?xml version="1.0" encoding="utf-8"?>
<navigation
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/fragment_item"
app:startDestination="@+id/menuHome">
<fragment
android:id="@+id/menuHome"
android:name="com.example.navigationview.HomeFragment"
android:label="Home"
tools:layout="@layout/fragment_home" />
<fragment
android:id="@+id/menuPerson"
android:name="com.example.navigationview.PersonFragment"
android:label="Person"
tools:layout="@layout/fragment_person" />
<fragment
android:id="@+id/menuWork"
android:name="com.example.navigationview.WorkFragment"
android:label="Work"
tools:layout="@layout/fragment_work" />
<fragment
android:id="@+id/menuTravel"
android:name="com.example.navigationview.TravelFragment"
android:label="Travel"
tools:layout="@layout/fragment_travel" />
</navigation>
The following code shows fragment_home.xml created in layout with a blank fragment. The blank fragment is created from com.example.navigationview> New > Fragment > Fragment (Blank) in java folder.
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".HomeFragment">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is Home fragment"
android:layout_gravity="center"
android:textSize="18sp"/>
</FrameLayout>
The following code shows fragment_person.xml created in layout with a blank fragment. The blank fragment is created from com.example.navigationview> New > Fragment > Fragment (Blank) in java folder.
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".PersonFragment">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is Person fragment"
android:layout_gravity="center"
android:textSize="18sp"/>
</FrameLayout>
The following code shows fragment_travel.xml created in layout with a blank fragment. The blank fragment is created from com.example.navigationview> New > Fragment > Fragment (Blank) in java folder.
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".TravelFragment">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is Travel fragment"
android:layout_gravity="center"
android:textSize="18sp"/>
</FrameLayout>
The following code shows fragment_work.xml created in layout with a blank fragment. The blank fragment is created from com.example.navigationview> New > Fragment > Fragment (Blank) in java folder.
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".WorkFragment">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is Work fragment"
android:layout_gravity="center"
android:textSize="18sp"/>
</FrameLayout>
The following code shows nav_menu.xml created in menu-(android resource directory) in res folder.
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/menuHome"
android:icon="@drawable/ic_baseline_home_24"
android:title="Home">
</item>
<item
android:id="@+id/menuPerson"
android:icon="@drawable/ic_baseline_person_24"
android:title="Person">
</item>
<item
android:id="@+id/menuWork"
android:icon="@drawable/ic_baseline_work_24"
android:title="Work">
</item>
<item
android:id="@+id/menuTravel"
android:icon="@drawable/ic_baseline_local_airport_24"
android:title="Travel">
</item>
</menu>
The following code shows nav_header_layout.xml created in layout of res folder.
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="18dp">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_baseline_wb_sunny_24"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
</ImageView>
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="15dp"
android:layout_marginLeft="47dp"
android:layout_marginTop="1dp"
android:text="Profile"
android:textSize="15sp"
android:textStyle="bold"
android:textColor="@color/teal_200"
app:layout_constraintStart_toEndOf="@+id/imageView"
app:layout_constraintTop_toTopOf="parent">
</TextView>
</androidx.constraintlayout.widget.ConstraintLayout>
Output:
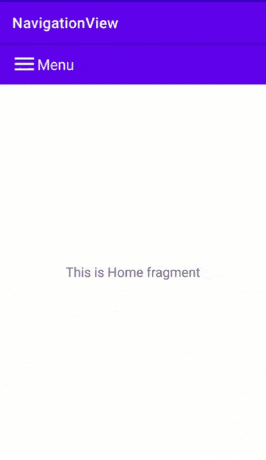